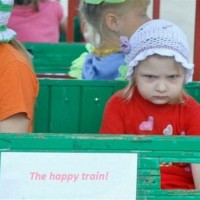
In my last post on clean coding I talked about why clean code was important, how it saves money in the long run and increases productivity. Now I want to talk a little bit about some of the do’s and don’ts of clean coding. Proper clean code without side effects takes discipline – extracting methods, classes, bundling parameters and local variables into DataObjects. The code, depending on how messy it started, might end up vastly different but the fact of the matter is when you start cleaning code you are sometimes restructuring thousands of lines and your ultimate goal is completely cosmetic. This is an important distinction, when you are finished cleaning up some code, it should do exactly what it did before you started. This is where you need the most discipline, even if you see glaring, obvious bugs, do not touch when you are cleaning.
With that in mind here are some major do's and don'ts.
Do’s:
- Get people on board. Clean code practices work best if everyone follows them, otherwise you are fighting an uphill battle. Once everyone is on board the happy train, you can see your codebase start to heal itself.
- Communicate. A developer does not want to start his IDE and not recognize what he is looking at. As a common courtesy and also to prevent major merging issues, let the developers know what you are cleaning up.
- Strive for commentless code. Sometimes comments are necessary, but if you need to put in a comment, you have probably failed at writing clean code.
- Rename for clarity. If you are looking at a variable and have no clue of its purpose based on the name, rename it so the purpose of the variable is clear. If the purpose of the variable cannot be expressed easily within a word or two, this is a sign the code is too complex.
- Inline or finalize the functions you are extracting. This is so you do not effect performance – having a method or function call added to the flow changes timing, slowing things down ever so slightly, but especially in time critical situations. Finalizing a method or in-lining a function will allow the compiler to completely mitigate any performance issues.
- Preserve scope. You will be extracting methods, extracting data classes, do not change the scope of the variables. Doing this changes the flow, causes side effects and will cause bugs.
- Use Data Classes. As you start extracting methods you may find the parameter list keeps growing, start putting those local variables and parameters into data classes. Methods with one parameter are acceptable, two is rarely justifiable, and anything more is not clean code.
- Turn large procedural methods/functions into their own classes. This makes the cleanup process much easier, allows you to turn all local variables within that method into fields within the new class (be aware of scope) keeping method parameters low.
- Unit test. You are now breaking up larger chunks of code into smaller, easier to test units. This is the confidence you need in making such drastic changes, more tests equals healthier code.
Don’ts
- Change the flow. Changing the flow of the code is the number one clean coding don’t. Once you change the flow, your changes stop being cosmetic and become dangerous. When you create bugs by changing the flow they become extremely difficult to fix.
- Leave commented out old code. This is what version control is for. Never comment out code in the clean code process, refactor, rename, remove redundancy and comments.
- Fix even the most obvious bugs. Make note, and talk to the original developer if possible to discuss the bug. However, under no circumstances should you fix that bug during a code clean up because you are breaking the cardinal rule, never change the flow. Once you are finished cleaning and confident the flow has not changed, feel free to fix away.
Keeping these do’s and don’ts in mind takes discipline but with practice, clean code will become second nature.